DBDriverSphinx Class Reference
[Sphinx]
DB Driver for Sphinx full text index. More...
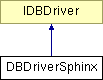
Public Member Functions |
|
escape ($value) | |
Escape given value. |
|
escape_database_entity ($obj, $type=self::FIELD) | |
Quote given database object, liek table,
field etc. |
|
execute ($sql) | |
Execute an SQL command (Insert, Update.
|
|
explain ($sql) | |
Explain the given query. |
|
get_db_name () | |
Returns name of DB. |
|
get_driver_name () | |
Return name of driver, e.g "mysql". |
|
get_host () | |
Returns host name of database. |
|
get_status () | |
Return current status. |
|
has_feature ($feature) | |
Returns true, if a given feature is
supported. |
|
initialize ($dbname, $user= '', $password= '', $host= 'localhost', $params=false) | |
Connect to DB. |
|
last_insert_id () | |
Get last insert ID. |
|
make_default () | |
Make this driver the default driver.
|
|
query ($query) | |
Execute a Select statement. |
|
quote ($value) | |
Quote given value. |
|
trans_commit () | |
Commit transaction. |
|
trans_rollback () | |
Rollback transaction. |
|
trans_start () | |
Start transaction. |
|
Static Public Member Functions |
|
static | strip_operators ($terms) |
Strip operators form searrch term. |
|
Public Attributes |
|
const | DEFAULT_CONNECTION_NAME = 'sphinx' |
const | FEATURE_MATCH_MODE = 'match_mode' |
Set matching mode. |
|
const | FEATURE_STRIP_OPERATORS = 'strip_operators' |
Set to strip operators: |
|
const | FEATURE_SUBINDEXES = 'subindexes' |
Query more than one index. |
|
const | FEATURE_WEIGHTS = 'weights' |
Set weights like so: |
|
const | MATCH_AND = 'and' |
AND all terms. |
|
const | MATCH_BOOL = 'bool' |
BOOLEAN match mode. |
|
const | MATCH_EX = 'ex' |
Extended mode, this is the default. |
|
const | MATCH_OR = 'or' |
OR all terms. |
|
const | MATCH_PHRASE = 'phrase' |
Match as phrase. |
|
const | SPHINX_ATTRIBUTE = 16384 |
Detailed Description
DB Driver for Sphinx full text index.
Definition at line 8 of file dbdriver.sphinx.php.
Member Function Documentation
DBDriverSphinx::escape | ( | $ | value | ) |
Escape given value.
- Parameters:
-
mixed $value
- Returns:
- string
Implements IDBDriver.
Definition at line 157 of file dbdriver.sphinx.php.
00157 { 00158 // Handcraft escaping for extended query syntax 00159 // TODO Maybe we should even leave it untouched within double quotes? 00160 $from = array ( '\\', '@', '&', '/'); 00161 $to = array ( '\\\\', '\@', '\&', '\/'); 00162 $ret = str_replace($from, $to, $value); 00163 00164 // This code escapes extended search operators like !, -, | etc. 00165 // That's definitely too much! 00166 //$this->connect(); 00167 //$ret = $this->client->EscapeString(Cast::string($value)); 00168 00169 return $ret; 00170 }
DBDriverSphinx::escape_database_entity | ( | $ | obj, | |
$ | type = self::FIELD |
|||
) |
Quote given database object, liek table, field etc.
- Parameters:
-
string $obj
Implements IDBDriver.
Definition at line 147 of file dbdriver.sphinx.php.
DBDriverSphinx::execute | ( | $ | sql | ) |
Execute an SQL command (Insert, Update.
..)
- Parameters:
-
string $sql
- Returns:
- Status
Implements IDBDriver.
Definition at line 193 of file dbdriver.sphinx.php.
DBDriverSphinx::explain | ( | $ | sql | ) |
Explain the given query.
- Parameters:
-
string $sql
- Returns:
- IDBResultSet False if quey cant be explain or driver does not support it
Implements IDBDriver.
Definition at line 297 of file dbdriver.sphinx.php.
DBDriverSphinx::get_db_name | ( | ) |
Returns name of DB.
- Returns:
- string
Implements IDBDriver.
Definition at line 97 of file dbdriver.sphinx.php.
00097 { 00098 return Arr::get_item($this->connect_params, 'dbname', ''); 00099 }
DBDriverSphinx::get_driver_name | ( | ) |
Return name of driver, e.g "mysql".
Lowercase!
- Returns:
- string
Implements IDBDriver.
Definition at line 75 of file dbdriver.sphinx.php.
DBDriverSphinx::get_host | ( | ) |
Returns host name of database.
- Returns:
- string
Implements IDBDriver.
Definition at line 84 of file dbdriver.sphinx.php.
00084 { 00085 $tmp = array( 00086 Arr::get_item($this->connect_params, 'host', ''), 00087 Arr::get_item($this->connect_params, 'port', '') 00088 ); 00089 return trim(implode($tmp), ':'); 00090 }
DBDriverSphinx::get_status | ( | ) |
Return current status.
- Returns:
- Status
Implements IDBDriver.
Definition at line 177 of file dbdriver.sphinx.php.
00177 { 00178 $this->connect(); 00179 $ret = new Status(); 00180 $err = $this->client->GetLastError(); 00181 if ($err) { 00182 $ret->append($err); 00183 } 00184 return $ret; 00185 }
DBDriverSphinx::has_feature | ( | $ | feature | ) |
Returns true, if a given feature is supported.
- Parameters:
-
string feature
- Returns:
- bool
Implements IDBDriver.
Definition at line 341 of file dbdriver.sphinx.php.
DBDriverSphinx::initialize | ( | $ | dbname, | |
$ | user = '' , |
|||
$ | password = '' , |
|||
$ | host =
'localhost' , |
|||
$ | params = false |
|||
) |
Connect to DB.
- Parameters:
-
string $dbname Ignored string $user Ignored string $password Ignored string $host Host
Implements IDBDriver.
Definition at line 109 of file dbdriver.sphinx.php.
00109 { 00110 $host_and_port = explode(':', $host); 00111 if (count($host_and_port) < 2) { 00112 $host_and_port[] = 9312; // Sphinx default port 00113 } 00114 $this->connect_params = array( 00115 'host' => $host_and_port[0], 00116 'port' => Cast::int($host_and_port[1]), 00117 'dbname' => $dbname 00118 ); 00119 }
DBDriverSphinx::last_insert_id | ( | ) |
DBDriverSphinx::make_default | ( | ) |
Make this driver the default driver.
- Returns:
- Status
Implements IDBDriver.
Definition at line 306 of file dbdriver.sphinx.php.
00306 { 00307 return new Status(); 00308 }
DBDriverSphinx::query | ( | $ | query | ) |
Execute a Select statement.
- Parameters:
-
string $sql
- Returns:
- IDBResultSet
Implements IDBDriver.
Definition at line 202 of file dbdriver.sphinx.php.
00202 { 00203 $this->connect(); 00204 00205 $arr_query = unserialize($query); 00206 $features = Arr::get_item($arr_query, 'features', false); 00207 00208 $terms = Arr::get_item_recursive($arr_query, 'conditions[query]', ''); 00209 if (Arr::get_item($features, self::FEATURE_STRIP_OPERATORS, false)) { 00210 $terms = self::strip_operators($terms); 00211 } 00212 00213 // Set options 00214 $this->client->SetSelect($arr_query['fields']); 00215 $this->client->SetArrayResult(true); 00216 if ($arr_query['order']) { 00217 $this->client->SetSortMode(SPH_SORT_EXTENDED, $arr_query['order']); 00218 } 00219 $limit = $arr_query['limit']; 00220 if ($limit) { 00221 $arr_limit = explode(';', $limit); 00222 $arr_limit = array_map('intval', $arr_limit); 00223 $this->client->SetLimits($arr_limit[0], $arr_limit[1]); 00224 } 00225 00226 // Filter 00227 foreach(Arr::get_item_recursive($arr_query, 'conditions[filter]', array()) as $filter) { 00228 $this->client->SetFilter( 00229 $filter['attribute'], 00230 $filter['values'], 00231 $filter['exclude'] 00232 ); 00233 } 00234 00235 // Field Weights 00236 $this->client->SetFieldWeights(Arr::get_item($features, self::FEATURE_WEIGHTS, array())); 00237 00238 // query 00239 $matchmode = ''; 00240 switch(Arr::get_item($features, self::FEATURE_MATCH_MODE, self::MATCH_EX)) { 00241 case self::MATCH_BOOL: 00242 $matchmode = SPH_MATCH_BOOLEAN; 00243 break; 00244 case self::MATCH_OR: 00245 $matchmode = SPH_MATCH_ANY; 00246 break; 00247 case self::MATCH_AND: 00248 $matchmode = SPH_MATCH_ALL; 00249 break; 00250 case self::MATCH_PHRASE: 00251 $matchmode = SPH_MATCH_PHRASE; 00252 break; 00253 case self::MATCH_EX: 00254 default: 00255 $matchmode = SPH_MATCH_EXTENDED2; 00256 break; 00257 } 00258 $this->client->SetMatchMode($matchmode); 00259 00260 // Fetch indexes 00261 $index_base_name = $this->connect_params['dbname'] . $arr_query['from']; 00262 $index_names = array(); 00263 $index_weight = array(); 00264 foreach(Arr::get_item($features, self::FEATURE_SUBINDEXES, array()) as $key => $value) { 00265 if (is_numeric($key)) { 00266 $subindex_name = $index_base_name . $value; 00267 } 00268 else { 00269 $subindex_name = $index_base_name . $key; 00270 $index_weight[$subindex_name] = $value; 00271 } 00272 $index_names[] = $subindex_name; 00273 } 00274 $index_name = count($index_names) ? implode(',', $index_names) : $index_base_name; 00275 if (count($index_weight)) { 00276 $this->client->SetIndexWeights($index_weight); 00277 } 00278 $result = $this->client->Query($terms, $index_name); 00279 00280 $ret = false; 00281 if (isset($features['count'])) { 00282 $ret = new DBResultSetCountSphinx($result, $this->get_status()); 00283 } 00284 else { 00285 $ret = new DBResultSetSphinx($result, $this->get_status()); 00286 } 00287 $this->client = false; 00288 return $ret; 00289 }
DBDriverSphinx::quote | ( | $ | value | ) |
Quote given value.
- Parameters:
-
string $value
Implements IDBDriver.
Definition at line 138 of file dbdriver.sphinx.php.
00138 { 00139 return "'" . $this->escape($value) . "'"; 00140 }
static DBDriverSphinx::strip_operators | ( | $ | terms | ) | [static] |
Strip operators form searrch term.
Definition at line 348 of file dbdriver.sphinx.php.
DBDriverSphinx::trans_commit | ( | ) |
DBDriverSphinx::trans_rollback | ( | ) |
DBDriverSphinx::trans_start | ( | ) |
Member Data Documentation
const DBDriverSphinx::DEFAULT_CONNECTION_NAME = 'sphinx' |
Definition at line 9 of file dbdriver.sphinx.php.
const DBDriverSphinx::FEATURE_MATCH_MODE = 'match_mode' |
Set matching mode.
$query->sphinx_features[DBDriverSphinx::FEATURE_MATCH_MODE] = DBDriverSphinx::MATCH_OR;
Definition at line 29 of file dbdriver.sphinx.php.
const DBDriverSphinx::FEATURE_STRIP_OPERATORS = 'strip_operators' |
Set to strip operators:
$query->sphinx_features[DBDriverSphinx::FEATURE_STRIP_OPERATORS] = true;
Definition at line 23 of file dbdriver.sphinx.php.
const DBDriverSphinx::FEATURE_SUBINDEXES = 'subindexes' |
Query more than one index.
$query->sphinx_features[DBDriverSphinx::FEATURE_SUBINDEXES] = array('main', 'delta'); $query->sphinx_features[DBDriverSphinx::FEATURE_SUBINDEXES] = array('main' => 1, 'delta' => 2);
If set, the given indexes are search. Note that naming rules still apply, that is the index name is build using APP_SPHINX_DB_NAME + table name + sub index name.
E.g. with a DB Name of "app_", a table named "seachindex" and two subindexes "main" and "delta", the indexes queried must be named "app_searchindexmain" and "app_searchindexdelta".
If an associative array is passed, the key is interpreted as subindex name and the value as weight. The data gets passed to Sphinx SetIndexWeights function
Definition at line 45 of file dbdriver.sphinx.php.
const DBDriverSphinx::FEATURE_WEIGHTS = 'weights' |
Set weights like so:
$query->sphinx_features[DBDriverSphinx::FEATURE_WEIGHTS] = array('column' => weight);
Definition at line 17 of file dbdriver.sphinx.php.
const DBDriverSphinx::MATCH_AND = 'and' |
AND all terms.
Definition at line 62 of file dbdriver.sphinx.php.
const DBDriverSphinx::MATCH_BOOL = 'bool' |
BOOLEAN match mode.
Definition at line 50 of file dbdriver.sphinx.php.
const DBDriverSphinx::MATCH_EX = 'ex' |
Extended mode, this is the default.
Definition at line 54 of file dbdriver.sphinx.php.
const DBDriverSphinx::MATCH_OR = 'or' |
OR all terms.
Definition at line 58 of file dbdriver.sphinx.php.
const DBDriverSphinx::MATCH_PHRASE = 'phrase' |
Match as phrase.
Definition at line 66 of file dbdriver.sphinx.php.
const DBDriverSphinx::SPHINX_ATTRIBUTE = 16384 |
Definition at line 10 of file dbdriver.sphinx.php.
The documentation for this class was generated from the following file:
- contributions/sphinx/model/drivers/sphinx/dbdriver.sphinx.php