PageViewBase Class Reference
[View]
Base class for rendering a whole page. More...
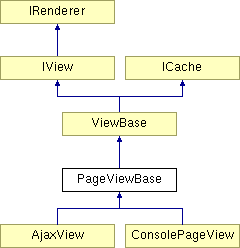
Public Member Functions |
|
__construct (PageData $page_data, $file=false) | |
render ($policy=self::NONE) | |
Process view and returnd the created
content. |
|
Public Attributes |
|
const | POLICY_GZIP = 1024 |
Protected Member Functions |
|
assign_default_vars ($policy) | |
Assign variables. |
|
do_render_cache ($cache, $policy) | |
Read content from cache object. |
|
format_error ($message) | |
Transform an error message into html.
|
|
get_cache_lifetime () | |
Returns cache life time in seconds. |
|
render_content (&$rendered_content, $policy) | |
Sets content. |
|
render_postprocess (&$rendered_content, $policy) | |
Called after content is rendered, always.
|
|
render_preprocess ($policy) | |
Called before content is rendered, always.
|
|
send_cache_headers ($lastmodified, $expires, $max_age=600, $etag= '') | |
Send cache control headers for cache.
|
|
send_status () | |
Send status. |
|
should_cache () | |
Returns true, if cache should be used.
|
|
store_cache ($cache_key, $content, $lifetime, $policy) | |
Write content to cache. |
|
Protected Attributes |
|
$cache_manager = null | |
$page_data = null |
Detailed Description
Base class for rendering a whole page.
Definition at line 10 of file pageviewbase.cls.php.
Constructor & Destructor Documentation
Definition at line 25 of file pageviewbase.cls.php.
00025 { 00026 $this->page_data = $page_data; 00027 $this->cache_manager = $page_data->get_cache_manager(); 00028 00029 if (empty($file)) { 00030 $file = 'page'; 00031 } 00032 parent::__construct($file, $this->cache_manager->get_cache_id()); 00033 }
Member Function Documentation
PageViewBase::assign_default_vars | ( | $ | policy | ) | [protected] |
Assign variables.
- Parameters:
-
$policy If set to IView::DISPLAY, content is printed, if false it is returned only
Reimplemented from ViewBase.
Reimplemented in AjaxView, and ConsolePageView.
Definition at line 212 of file pageviewbase.cls.php.
00212 { 00213 parent::assign_default_vars($policy); 00214 00215 // Check for error templates and render error content, if required 00216 switch ($this->page_data->status_code) { 00217 case CONTROLLER_ACCESS_DENIED: 00218 case CONTROLLER_NOT_FOUND: 00219 case CONTROLLER_INTERNAL_ERROR: 00220 $error_view = ViewFactory::create_view( 00221 IViewFactory::CONTENT, 00222 'errors/' . String::plain_ascii($this->page_data->status_code, '_'), 00223 $this->page_data 00224 ); 00225 $this->page_data->head->robots_index = ROBOTS_NOINDEX_FOLLOW; 00226 $error_view->render(); 00227 break; 00228 default: 00229 break; 00230 } 00231 00232 $this->page_data->sort_blocks(); 00233 $this->assign('page_data', $this->page_data); 00234 $this->assign('pagetitle', $this->page_data->head->title); 00235 $this->assign('pagedescr', String::substr_word($this->page_data->head->description, 0, 200)); 00236 $this->assign('status', $this->page_data->status); 00237 $this->assign('blocks', $this->page_data->blocks); 00238 $this->assign('content', $this->page_data->content); 00239 $breadcrumb = is_string($this->page_data->breadcrumb) ? $this->page_data->breadcrumb : WidgetBreadcrumb::output($this->page_data->breadcrumb); 00240 if (Config::get_value(Config::VERSION_MAX) < 0.6) { 00241 // In 0.5 PageData::breadcrumb is alwys the result of WidgetBreadcrumb 00242 $this->page_data->breadcrumb = $breadcrumb; 00243 } 00244 $this->assign('breadcrumb', $breadcrumb); 00245 00246 if ($this->page_data->router) { 00247 $this->assign('route_id', $this->page_data->router->get_route_id()); 00248 } 00249 }
PageViewBase::do_render_cache | ( | $ | cache, | |
$ | policy | |||
) | [protected] |
Read content from cache object.
- Parameters:
-
$policy If set to IView::DISPLAY, content is printed, if false it is returned only $cache ICacheItem instance
- Returns:
- mixed
Reimplemented from ViewBase.
Definition at line 169 of file pageviewbase.cls.php.
00169 { 00170 $ret = ''; 00171 if ($cache) { 00172 $cache_data = $cache->get_data(); 00173 foreach(Arr::get_item($cache_data, 'headers', array()) as $header) { 00174 GyroHeaders::set($header, false, true); 00175 } 00176 //$etag = Arr::get_item($cache_data, 'etag', ''); 00177 $cache_header_manager = Arr::get_item($cache_data, 'cacheheadermanager', $this->cache_manager->get_cache_header_manager()); 00178 $this->cache_manager->set_cache_header_manager($cache_header_manager); 00179 $this->page_data->status_code = Arr::get_item($cache_data, 'status', ''); 00180 $this->page_data->in_history = Arr::get_item($cache_data, 'in_history', true); 00181 if (Common::flag_is_set($policy, self::POLICY_GZIP)) { 00182 $ret = $cache->get_content_compressed(); 00183 } 00184 else { 00185 $ret = $cache->get_content_plain(); 00186 } 00187 } 00188 return $ret; 00189 }
PageViewBase::format_error | ( | $ | message | ) | [protected] |
Transform an error message into html.
- Parameters:
-
$message Error message as string
- Returns:
- string
Definition at line 257 of file pageviewbase.cls.php.
00257 { 00258 return html::error($message); 00259 }
PageViewBase::get_cache_lifetime | ( | ) | [protected] |
Returns cache life time in seconds.
- Returns:
- int
Reimplemented from ViewBase.
Definition at line 122 of file pageviewbase.cls.php.
PageViewBase::render | ( | $ | policy =
self::NONE |
) |
Process view and returnd the created content.
- Parameters:
-
$policy If set to IView::DISPLAY, content is printed, if false it is returned only
- Returns:
- string The rendered content
Reimplemented from ViewBase.
Reimplemented in ConsolePageView.
Definition at line 41 of file pageviewbase.cls.php.
00041 { 00042 if (Config::has_feature(Config::GZIP_SUPPORT)) { 00043 $policy |= self::POLICY_GZIP; 00044 } 00045 return parent::render($policy); 00046 }
PageViewBase::render_content | ( | &$ | rendered_content, | |
$ | policy | |||
) | [protected] |
Sets content.
- Parameters:
-
$rendered_content The content rendered $policy If set to IView::DISPLAY, content is printed, if false it is returned only
- Returns:
- void
Reimplemented from ViewBase.
Reimplemented in AjaxView.
Definition at line 69 of file pageviewbase.cls.php.
00069 { 00070 parent::render_content($rendered_content, $policy); 00071 if (Common::flag_is_set($policy, self::POLICY_GZIP)) { 00072 $rendered_content = gzdeflate($rendered_content, 9); 00073 } 00074 }
PageViewBase::render_postprocess | ( | &$ | rendered_content, | |
$ | policy | |||
) | [protected] |
Called after content is rendered, always.
- Parameters:
-
$rendered_content The content rendered $policy If set to IView::DISPLAY, content is printed, if false it is returned only
- Returns:
- void
Reimplemented from ViewBase.
Reimplemented in AjaxView, and ConsolePageView.
Definition at line 83 of file pageviewbase.cls.php.
00083 { 00084 if (!Common::flag_is_set($policy, self::CONTENT_ONLY)) { 00085 $this->send_status(); 00086 $cache_header_manager = $this->cache_manager->get_cache_header_manager(); 00087 $cache_header_manager->send_headers( 00088 $rendered_content, 00089 $this->cache_manager->get_expiration_datetime(), 00090 $this->cache_manager->get_creation_datetime() 00091 ); 00092 00093 if (Common::flag_is_set($policy, self::POLICY_GZIP)) { 00094 GyroHeaders::set('Content-Encoding', 'deflate', true); 00095 } 00096 GyroHeaders::set('Vary', 'Accept-Encoding', false); 00097 GyroHeaders::set('Date', GyroDate::http_date(time()), true); 00098 00099 GyroHeaders::send(); 00100 } 00101 }
PageViewBase::render_preprocess | ( | $ | policy | ) | [protected] |
Called before content is rendered, always.
- Parameters:
-
$policy If set to IView::DISPLAY, content is printed, if false it is returned only
- Returns:
- void
Reimplemented from ViewBase.
Reimplemented in ConsolePageView.
Definition at line 54 of file pageviewbase.cls.php.
00054 { 00055 parent::render_preprocess($policy); 00056 // Change Template Path 00057 if (!empty($this->page_data->page_template)) { 00058 $this->template = $this->page_data->page_template; 00059 } 00060 }
PageViewBase::send_cache_headers | ( | $ | lastmodified, | |
$ | expires, | |||
$ | max_age = 600 , |
|||
$ | etag = '' |
|||
) | [protected] |
Send cache control headers for cache.
- Parameters:
-
$lastmodified A timestamp $expires A timestamp $max_age Max age in seconds
Definition at line 198 of file pageviewbase.cls.php.
00198 { 00199 $max_age = intval($max_age); 00200 GyroHeaders::set('Pragma', '', false); 00201 GyroHeaders::set('Cache-Control', "private, must-revalidate, max-age=$max_age", false); 00202 GyroHeaders::set('Last-Modified', GyroDate::http_date($lastmodified), false); 00203 GyroHeaders::set('Expires', GyroDate::http_date($expires), false); 00204 GyroHeaders::set('Etag', $etag, true); 00205 }
PageViewBase::send_status | ( | ) | [protected] |
Send status.
Definition at line 264 of file pageviewbase.cls.php.
00264 { 00265 $log = Config::has_feature(Config::LOG_HTML_ERROR_STATUS); 00266 switch ($this->page_data->status_code) { 00267 case CONTROLLER_ACCESS_DENIED: 00268 Common::send_status_code(403); // Forbidden 00269 break; 00270 case CONTROLLER_NOT_FOUND: 00271 Common::send_status_code(404); //Not found 00272 break; 00273 case CONTROLLER_INTERNAL_ERROR: 00274 Common::send_status_code(503); // Service unavailable 00275 break; 00276 default: 00277 // OK, a valid page. This can be remembered, if allowed 00278 if ($this->page_data->in_history) { 00279 History::push(Url::current()); 00280 } 00281 $log = false; 00282 break; 00283 } 00284 if ($log) { 00285 Load::components('referer', 'logger'); 00286 $referer = Referer::current(); 00287 $request = RequestInfo::current(); 00288 $params = array( 00289 'code' => $this->page_data->status_code, 00290 'referer' => $referer->build(), 00291 'referer_org' => $referer->get_original_referer_url(), 00292 'useragent' => $request->user_agent(), 00293 'host' => $request->remote_host() 00294 ); 00295 Logger::log('html_error_status', $params); 00296 } 00297 }
PageViewBase::should_cache | ( | ) | [protected] |
Returns true, if cache should be used.
- Returns:
- bool
Reimplemented from ViewBase.
Reimplemented in AjaxView, and ConsolePageView.
Definition at line 108 of file pageviewbase.cls.php.
00108 { 00109 $ret = parent::should_cache(); 00110 if ($ret) { 00111 // Do not read cache, if a message should be or has been displayed 00112 $ret = empty($this->page_data->status) || $this->page_data->status->is_empty(); 00113 } 00114 return $ret; 00115 }
PageViewBase::store_cache | ( | $ | cache_key, | |
$ | content, | |||
$ | lifetime, | |||
$ | policy | |||
) | [protected] |
Write content to cache.
- Parameters:
-
$cache_key $content $lifetime
Reimplemented from ViewBase.
Definition at line 133 of file pageviewbase.cls.php.
00133 { 00134 $headers = array(); 00135 $forbidden = array( 00136 'age', 00137 'date', 00138 'content-encoding', 00139 'content-length', 00140 'server', 00141 'set-cookie', 00142 'transfer-encoding', 00143 'x-powered-by', 00144 'keep-alive', 00145 'connection' 00146 ); 00147 foreach(GyroHeaders::headers() as $name => $val) { 00148 if (!in_array($name, $forbidden)) { 00149 $headers[] = $val; 00150 } 00151 } 00152 $cache_data = array( 00153 'status' => $this->page_data->status_code, 00154 'in_history' => $this->page_data->in_history, 00155 'headers' => $headers, 00156 'cacheheadermanager' => $this->cache_manager->get_cache_header_manager() 00157 ); 00158 $gziped = Common::flag_is_set($policy, self::POLICY_GZIP); 00159 Cache::store($cache_key, $content, $lifetime, $cache_data, $gziped); 00160 }
Member Data Documentation
PageViewBase::$cache_manager = null
[protected] |
Definition at line 16 of file pageviewbase.cls.php.
PageViewBase::$page_data = null
[protected] |
Definition at line 23 of file pageviewbase.cls.php.
const PageViewBase::POLICY_GZIP = 1024 |
Definition at line 11 of file pageviewbase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/view/base/pageviewbase.cls.php