MailBaseCommand Class Reference
[Behaviour]
Generic class for sending a mail. More...
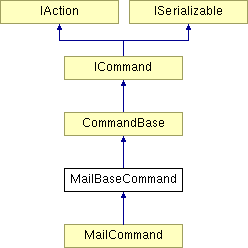
Public Member Functions |
|
__construct ($subject, $email, $template, $template_args=array()) | |
Constructor. |
|
add_attachment ($file_name, $name= '') | |
Add an attachment. |
|
can_execute ($user) | |
Returns TRUE, if the command can be executed
by given user. |
|
execute () | |
Send Mail. |
|
get_alt_message () | |
Get alternative message. |
|
set_alt_message ($alt_message) | |
Set alternative message. |
|
set_is_html ($yesno) | |
Set if this is a HTML mail or not. |
|
set_subject ($subject) | |
Create Mail Subject. |
|
Protected Member Functions |
|
compute_alt_message ($org_message) | |
Compute the alternate message. |
|
create_mail_message ($subject, $message, $to) | |
Create the mail message instance. |
|
get_attachments () | |
Returns array of filenames to attach.
|
|
get_message () | |
Return mail message. |
|
get_message_extension () | |
Returns default text, that gets appends to
every mail. |
|
get_subject () | |
Create Mail Subject. |
|
get_to () | |
Create Mail Recipient. |
|
is_html () | |
Returns wether this is a HTML mail or not.
|
|
set_error ($message) | |
Set an error message. |
|
Protected Attributes |
|
$alt_message = '' | |
$email = '' | |
Email address. |
|
$err = null | |
Error object. |
|
$files_to_attach = array() | |
$html_mail = false | |
HTML or not? |
|
$subject | |
Subject of mail. |
|
$template = null | |
The name of the template. |
|
$template_args = false | |
Asscoiative array of template arguments.
|
|
$view |
Detailed Description
Generic class for sending a mail.
The class gets passed a template which then is rendered. Within the template the command itself is available as $mailcmd.
The template may set title, content-type, alternate messages and attachments.
Subclasses can be specialized to provide attachments and a mail footer for every mail.
HTML Mail
A template may contain HTML. If so, it should call
$mailcmd->set_is_html(true);
HTML should have a plain text fallback. The easies ways to achieve this, is to pass the view itself, which will autmatically get converted to plain text:
$mailcmd->set_alt_message($self);
Definition at line 32 of file mail.cmd.php.
Constructor & Destructor Documentation
MailBaseCommand::__construct | ( | $ | subject, | |
$ | email, | |||
$ | template, | |||
$ | template_args =
array() |
|||
) |
Constructor.
- Parameters:
-
String Subject of Mail String Mail address String Template name Array Associative array of template arguments
Definition at line 64 of file mail.cmd.php.
00064 { 00065 $this->template = $template; 00066 $this->template_args = $template_args; 00067 $this->subject = $subject; 00068 $this->email = $email; 00069 00070 $this->err = new Status(); 00071 }
Member Function Documentation
MailBaseCommand::add_attachment | ( | $ | file_name, | |
$ | name = '' |
|||
) |
Add an attachment.
- Parameters:
-
string $file_name Absolute path to file string $name Optional name of attachment
Definition at line 258 of file mail.cmd.php.
MailBaseCommand::can_execute | ( | $ | user | ) |
Returns TRUE, if the command can be executed by given user.
Reimplemented from CommandBase.
Definition at line 73 of file mail.cmd.php.
MailBaseCommand::compute_alt_message | ( | $ | org_message | ) | [protected] |
Compute the alternate message.
- Parameters:
-
string $org_message Original message. Converted to plain text, if the alt message is the current view
Definition at line 137 of file mail.cmd.php.
00137 { 00138 $ret = ''; 00139 if ($this->alt_message instanceof IView) { 00140 if ($this->alt_message == $this->view) { 00141 $ret = $org_message; // Prevent view from beeing rendered twice 00142 if ($this->is_html()) { 00143 $ret = ConverterFactory::decode($ret, ConverterFactory::HTML_EX, array('p' => "\n\n", 'a' => '$url$')); 00144 } 00145 } else { 00146 $ret = $this->alt_message->render(); 00147 } 00148 } 00149 return $ret; 00150 }
MailBaseCommand::create_mail_message | ( | $ | subject, | |
$ | message, | |||
$ | to | |||
) | [protected] |
Create the mail message instance.
- Returns:
- MailMessage
Definition at line 127 of file mail.cmd.php.
00127 { 00128 $content_type = $this->html_mail ? 'text/html; charset=%charset' : ''; 00129 return new MailMessage($subject, $message, $to, '', $content_type); 00130 }
MailBaseCommand::execute | ( | ) |
Send Mail.
Implements a template method and calls functions for subject, from, and template
Reimplemented from CommandBase.
Definition at line 85 of file mail.cmd.php.
00085 { 00086 $to = $this->get_to(); 00087 if (empty($to)) { 00088 return $this->err; // No recipient, no mail... 00089 } 00090 00091 $message = $this->get_message(); 00092 $append = $this->get_message_extension(); 00093 if (!empty($append)) { 00094 $message .= "\n\n------------------------\n\n" . $append; 00095 } 00096 00097 $subject = $this->get_subject(); 00098 00099 if ($this->err->is_ok()) { 00100 $mail = $this->create_mail_message($subject, $message, $to); 00101 00102 // Set alternative message 00103 $mail->set_alt_message($this->compute_alt_message($message)); 00104 00105 // Set custom attachments 00106 foreach($this->files_to_attach as $filename => $name) { 00107 $mail->add_attachment($filename, $name); 00108 } 00109 00110 // Set overlaoded attachments 00111 foreach(Arr::force($this->get_attachments(), false) as $a) { 00112 $mail->add_attachment($a); 00113 } 00114 00115 // Send 00116 $this->err->merge($mail->send()); 00117 } 00118 00119 return $this->err; 00120 }
MailBaseCommand::get_alt_message | ( | ) |
MailBaseCommand::get_attachments | ( | ) | [protected] |
Returns array of filenames to attach.
Definition at line 208 of file mail.cmd.php.
MailBaseCommand::get_message | ( | ) | [protected] |
Return mail message.
Definition at line 176 of file mail.cmd.php.
00176 { 00177 $this->view = ViewFactory::create_view(IViewFactory::MESSAGE, $this->template); 00178 if ($this->view) { 00179 foreach(Arr::force($this->template_args) as $name => $value) { 00180 $this->view->assign($name, $value); 00181 } 00182 $this->view->assign('to', $this->get_to()); 00183 $this->view->assign('mailcmd', $this); 00184 return $this->view->render(); 00185 } 00186 else { 00187 $this->set_error(tr('Mail message view not created', 'commands')); 00188 } 00189 }
MailBaseCommand::get_message_extension | ( | ) | [protected] |
Returns default text, that gets appends to every mail.
Definition at line 194 of file mail.cmd.php.
MailBaseCommand::get_subject | ( | ) | [protected] |
Create Mail Subject.
Definition at line 169 of file mail.cmd.php.
MailBaseCommand::get_to | ( | ) | [protected] |
Create Mail Recipient.
Definition at line 201 of file mail.cmd.php.
00201 { 00202 return Cast::string($this->email); 00203 }
MailBaseCommand::is_html | ( | ) | [protected] |
Returns wether this is a HTML mail or not.
- Returns:
- bool
Definition at line 230 of file mail.cmd.php.
MailBaseCommand::set_alt_message | ( | $ | alt_message | ) |
Set alternative message.
- Parameters:
-
string $alt_message
Definition at line 239 of file mail.cmd.php.
00239 { 00240 $this->alt_message = $alt_message; 00241 }
MailBaseCommand::set_error | ( | $ | message | ) | [protected] |
Set an error message.
Definition at line 155 of file mail.cmd.php.
MailBaseCommand::set_is_html | ( | $ | yesno | ) |
Set if this is a HTML mail or not.
Can be set through template:
$mailcmd->set_is_html(true);
Definition at line 221 of file mail.cmd.php.
MailBaseCommand::set_subject | ( | $ | subject | ) |
Create Mail Subject.
Definition at line 162 of file mail.cmd.php.
00162 { 00163 $this->subject = $subject; 00164 }
Member Data Documentation
MailBaseCommand::$alt_message = ''
[protected] |
Definition at line 53 of file mail.cmd.php.
MailBaseCommand::$email = ''
[protected] |
Email address.
Definition at line 40 of file mail.cmd.php.
MailBaseCommand::$err = null
[protected] |
Error object.
Definition at line 45 of file mail.cmd.php.
MailBaseCommand::$files_to_attach = array()
[protected] |
Definition at line 54 of file mail.cmd.php.
MailBaseCommand::$html_mail = false
[protected] |
HTML or not?
Definition at line 42 of file mail.cmd.php.
MailBaseCommand::$subject
[protected] |
Subject of mail.
Definition at line 38 of file mail.cmd.php.
MailBaseCommand::$template = null
[protected] |
The name of the template.
Definition at line 34 of file mail.cmd.php.
MailBaseCommand::$template_args = false
[protected] |
Asscoiative array of template arguments.
Definition at line 36 of file mail.cmd.php.
MailBaseCommand::$view
[protected] |
Definition at line 51 of file mail.cmd.php.
The documentation for this class was generated from the following file:
- gyro/core/behaviour/commands/base/mail.cmd.php