DBSqlBuilderSelect Class Reference
[Model]
Build a select query. More...
Inheritance diagram for
DBSqlBuilderSelect:
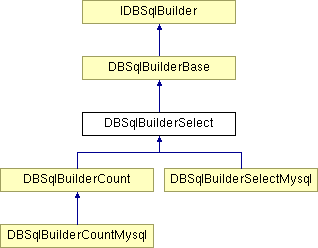
Protected Member Functions |
|
get_fieldnames ($arr_fields, IDBTable $table) | |
get_group_by ($arr_group_by) | |
get_having (IDBWhere $having) | |
get_join ($arr_subqueries) | |
get_single_join (DBQueryJoined $joined_query) | |
get_sql_template () | |
get_substitutes () |
Detailed Description
Build a select query.
Definition at line 8 of file dbsqlbuilder.select.cls.php.
Member Function Documentation
DBSqlBuilderSelect::get_fieldnames | ( | $ | arr_fields, | |
IDBTable $ | table | |||
) | [protected] |
Reimplemented in DBSqlBuilderCount.
Definition at line 30 of file dbsqlbuilder.select.cls.php.
00030 { 00031 $ret = ''; 00032 $connection = $table->get_table_driver(); 00033 $fieldnames = array(); 00034 if (count($arr_fields) == 0) { 00035 $arr_fields = array_keys($table->get_table_fields()); 00036 } 00037 foreach($arr_fields as $key => $name) { 00038 $has_alias = !is_numeric($key); 00039 $fieldname = $has_alias ? $key : $name; 00040 $fieldalias = $name; //String::plain_ascii($name, '', true); 00041 00042 $dbfield = $table->get_table_field($fieldname); 00043 $statement = ($dbfield) ? $dbfield->format_select() : $fieldname; 00044 $statement = str_replace($fieldname, $this->prefix_column($fieldname, $table), $statement); 00045 if ($fieldalias != '*') { 00046 $statement .= ' AS ' . DB::escape_database_entity($fieldalias, $connection, IDBDriver::ALIAS); 00047 } 00048 00049 $fieldnames[] = $statement; 00050 } 00051 if (count($fieldnames)) { 00052 $ret = implode(', ', $fieldnames); 00053 } 00054 else { 00055 $ret = $this->prefix_column('*', $table); 00056 } 00057 return $ret; 00058 }
DBSqlBuilderSelect::get_group_by | ( | $ | arr_group_by | ) | [protected] |
Definition at line 92 of file dbsqlbuilder.select.cls.php.
00092 { 00093 $ret = ''; 00094 if (count($arr_group_by) > 0) { 00095 $ret .= ' GROUP BY '; 00096 $items = array(); 00097 foreach($arr_group_by as $group_by) { 00098 $column = Arr::get_item($group_by, 'field', ''); 00099 if (empty($column)) { 00100 continue; 00101 } 00102 $table = Arr::get_item($group_by, 'table', null); 00103 $items[] = $column; 00104 } 00105 $ret .= implode(', ', $items); 00106 } 00107 return $ret; 00108 }
DBSqlBuilderSelect::get_having | ( | IDBWhere $ | having | ) | [protected] |
Definition at line 110 of file dbsqlbuilder.select.cls.php.
DBSqlBuilderSelect::get_join | ( | $ | arr_subqueries | ) | [protected] |
Definition at line 60 of file dbsqlbuilder.select.cls.php.
00060 { 00061 $ret = ''; 00062 foreach($arr_subqueries as $subquery) { 00063 $ret .= $this->get_single_join($subquery); 00064 } 00065 return $ret; 00066 }
DBSqlBuilderSelect::get_single_join | ( | DBQueryJoined $ | joined_query | ) | [protected] |
Definition at line 68 of file dbsqlbuilder.select.cls.php.
00068 { 00069 $ret = ' '; 00070 switch ($joined_query->get_join_type()) { 00071 case DBQueryJoined::LEFT: 00072 $ret .= 'LEFT JOIN'; 00073 break; 00074 case DBQueryJoined::RIGHT: 00075 $ret .= 'RIGHT JOIN'; 00076 break; 00077 default: 00078 $ret .= 'INNER JOIN'; 00079 break; 00080 } 00081 $ret .= ' '; 00082 $ret .= $this->get_table_and_alias($joined_query->get_table()); 00083 $ret .= ' ON '; 00084 $ret .= $joined_query->get_join_conditions()->get_sql(); 00085 00086 // Recursive.. 00087 $ret .= $this->get_join($joined_query->get_subqueries()); 00088 00089 return $ret; 00090 }
DBSqlBuilderSelect::get_sql_template | ( | ) | [protected] |
Reimplemented from DBSqlBuilderBase.
Reimplemented in DBSqlBuilderCount.
Definition at line 9 of file dbsqlbuilder.select.cls.php.
DBSqlBuilderSelect::get_substitutes | ( | ) | [protected] |
Reimplemented from DBSqlBuilderBase.
Reimplemented in DBSqlBuilderCount.
Definition at line 13 of file dbsqlbuilder.select.cls.php.
00013 { 00014 $table = $this->query->get_table(); 00015 $ret = array( 00016 '%!fields' => $this->get_fieldnames($this->fields, $table), 00017 '%!from' => $this->get_table_and_alias($table), 00018 '%where' => $this->get_where($this->query->get_wheres()), 00019 '%join' => $this->get_join($this->query->get_subqueries()), 00020 '%distinct' => $this->get_feature_sql($this->params, 'distinct', 'DISTINCT'), 00021 '%for_update' => $this->get_feature_sql($this->params, 'for_update', 'FOR UPDATE'), 00022 '%group_by' => $this->get_group_by(Arr::get_item($this->params, 'group_by', array())), 00023 '%having' => $this->get_having($this->query->get_havings()), 00024 '%limit' => $this->get_limit(Arr::get_item($this->params, 'limit', array(0,0))), 00025 '%order_by' => $this->get_order_by(Arr::get_item($this->params, 'order_by', array())) 00026 ); 00027 return $ret; 00028 }
The documentation for this class was generated from the following file:
- gyro/core/model/base/sqlbuilder/dbsqlbuilder.select.cls.php