DBField Class Reference
[Model]
Base class to represent a field. More...
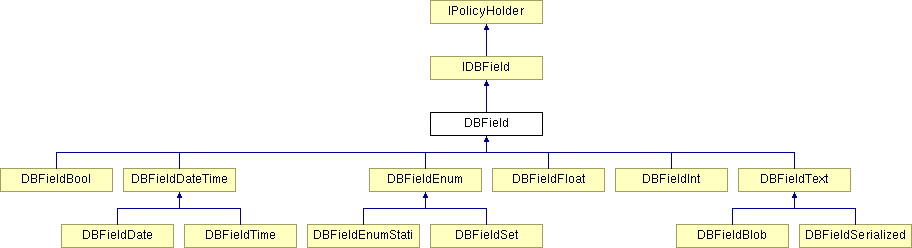
Public Member Functions |
|
__construct ($name, $default_value=null, $policy=self::NONE, $connection=DB::DEFAULT_CONNECTION) | |
convert_result ($value) | |
Transform result from SELECT to native.
|
|
format ($value) | |
Reformat passed value to DB
format. |
|
format_select () | |
Allow replacements for field in select from
clause. |
|
format_where ($value) | |
Format for use in WHERE clause. |
|
get_field_default () | |
Returns the default value for this field.
|
|
get_field_name () | |
Returns name. |
|
get_null_allowed () | |
Returns true, if null values are allowed.
|
|
get_policy () | |
Return policy. |
|
get_table () | |
Get table field belongs to. |
|
has_default_value () | |
Returns true, if field has default value.
|
|
has_policy ($policy) | |
Returns true, if client has given policy.
|
|
read_from_array ($arr) | |
Reads value from array (e.g $_POST) and
converts it into something meaningfull. |
|
set_connection ($connection) | |
Set connection of field. |
|
set_policy ($policy) | |
Set policy. |
|
set_table ($table) | |
Set table field belongs to. |
|
validate ($value) | |
Returns true, if the value passed fits the
fields restrictions. |
|
Public Attributes |
|
const | INTERNAL = 8 |
Indicates this field is internal. |
|
const | NOT_NULL = 1 |
Indicates this field may not be NULL.
|
|
Protected Member Functions |
|
do_format_not_null ($value) | |
Format values that are not NULL. |
|
do_format_null ($value) | |
Format values that are NULL. |
|
get_connection () | |
Returns connection. |
|
get_field_name_translation () | |
Translate field name. |
|
is_null ($value) | |
Returns true if $value is NULL or DBNull.
|
|
quote ($value) | |
Quote value. |
|
Protected Attributes |
|
$connection | |
$default_value = null | |
$name = '' | |
$policy | |
$table |
Detailed Description
Base class to represent a field.
Definition at line 8 of file dbfield.cls.php.
Constructor & Destructor Documentation
DBField::__construct | ( | $ | name, | |
$ | default_value =
null , |
|||
$ | policy =
self::NONE , |
|||
$ | connection = DB::DEFAULT_CONNECTION |
|||
) |
Reimplemented in DBFieldEnum, DBFieldSerialized, and DBFieldText.
Definition at line 49 of file dbfield.cls.php.
00049 { 00050 $this->name = $name; 00051 $this->default_value = $default_value; 00052 $this->policy = $policy; 00053 $this->connection = $connection; 00054 }
Member Function Documentation
DBField::convert_result | ( | $ | value | ) |
Transform result from SELECT to native.
- Parameters:
-
mixed $value
- Returns:
- mixed
Implements IDBField.
Reimplemented in DBFieldBool, DBFieldDateTime, DBFieldSerialized, and DBFieldSet.
Definition at line 178 of file dbfield.cls.php.
DBField::do_format_not_null | ( | $ | value | ) | [protected] |
Format values that are not NULL.
- Parameters:
-
mixed $value
- Returns:
- string
Reimplemented in DBFieldBlob, DBFieldBool, DBFieldFloat, DBFieldInt, DBFieldSerialized, and DBFieldSet.
Definition at line 151 of file dbfield.cls.php.
00151 { 00152 return $this->quote($value); 00153 }
DBField::do_format_null | ( | $ | value | ) | [protected] |
Format values that are NULL.
- Parameters:
-
mixed $value
- Returns:
- string
Definition at line 141 of file dbfield.cls.php.
DBField::format | ( | $ | value | ) |
Reformat passed value to DB format.
- Parameters:
-
mixed $value
- Returns:
- string
Implements IDBField.
Reimplemented in DBFieldDateTime.
Definition at line 126 of file dbfield.cls.php.
00126 { 00127 if ($this->is_null($value)) { 00128 return $this->do_format_null($value); 00129 } 00130 else { 00131 return $this->do_format_not_null($value); 00132 } 00133 }
DBField::format_select | ( | ) |
Allow replacements for field in select from clause.
Implements IDBField.
Reimplemented in DBFieldBool, DBFieldDateTime, DBFieldSet, and DBFieldTime.
Definition at line 168 of file dbfield.cls.php.
00168 { 00169 return $this->get_field_name(); 00170 }
DBField::format_where | ( | $ | value | ) |
Format for use in WHERE clause.
- Parameters:
-
mixed $value
- Returns:
- string
Implements IDBField.
Reimplemented in DBFieldDateTime.
Definition at line 161 of file dbfield.cls.php.
00161 { 00162 return $this->format($value); 00163 }
DBField::get_connection | ( | ) | [protected] |
DBField::get_field_default | ( | ) |
Returns the default value for this field.
- Returns:
- mixed
Implements IDBField.
Reimplemented in DBFieldDateTime, DBFieldInt, and DBFieldSerialized.
Definition at line 70 of file dbfield.cls.php.
DBField::get_field_name | ( | ) |
DBField::get_field_name_translation | ( | ) | [protected] |
DBField::get_null_allowed | ( | ) |
Returns true, if null values are allowed.
- Returns:
- bool
Implements IDBField.
Definition at line 86 of file dbfield.cls.php.
00086 { 00087 return !Common::flag_is_set($this->policy, self::NOT_NULL); 00088 }
DBField::get_policy | ( | ) |
Return policy.
- Returns:
- int
Implements IPolicyHolder.
Definition at line 233 of file dbfield.cls.php.
DBField::get_table | ( | ) |
DBField::has_default_value | ( | ) |
Returns true, if field has default value.
Implements IDBField.
Reimplemented in DBFieldInt.
Definition at line 77 of file dbfield.cls.php.
00077 { 00078 return !$this->is_null($this->default_value); 00079 }
DBField::has_policy | ( | $ | policy | ) |
Returns true, if client has given policy.
- Parameters:
-
int $policy
- Returns:
- bool
Implements IPolicyHolder.
Definition at line 252 of file dbfield.cls.php.
00252 { 00253 $ret = Common::flag_is_set($this->policy, $policy); 00254 return $ret; 00255 }
DBField::is_null | ( | $ | value | ) | [protected] |
Returns true if $value is NULL or DBNull.
Definition at line 267 of file dbfield.cls.php.
DBField::quote | ( | $ | value | ) | [protected] |
Quote value.
Definition at line 260 of file dbfield.cls.php.
00260 { 00261 return DB::quote(Cast::string($value), $this->get_connection()); 00262 }
DBField::read_from_array | ( | $ | arr | ) |
Reads value from array (e.g $_POST) and converts it into something meaningfull.
Implements IDBField.
Reimplemented in DBFieldBool, and DBFieldFloat.
Definition at line 185 of file dbfield.cls.php.
00185 { 00186 return Arr::get_item($arr, $this->get_field_name(), null); 00187 }
DBField::set_connection | ( | $ | connection | ) |
Set connection of field.
- Parameters:
-
string|IDBDriver $connection
- Returns:
- void
Implements IDBField.
Definition at line 195 of file dbfield.cls.php.
00195 { 00196 $this->connection = $connection; 00197 }
DBField::set_policy | ( | $ | policy | ) |
Set policy.
- Parameters:
-
int $policy
Implements IPolicyHolder.
Definition at line 242 of file dbfield.cls.php.
00242 { 00243 $this->policy = $policy; 00244 }
DBField::set_table | ( | $ | table | ) |
Set table field belongs to.
- Attention:
- The table may not be set, fields must be aware of this!
- Parameters:
-
IDBTable $table
- Returns:
- void
Implements IDBField.
Definition at line 215 of file dbfield.cls.php.
00215 { 00216 $this->table = $table; 00217 }
DBField::validate | ( | $ | value | ) |
Returns true, if the value passed fits the fields restrictions.
- Parameters:
-
mixed $value
- Returns:
- Status
Implements IDBField.
Reimplemented in DBFieldBlob, DBFieldDateTime, DBFieldEnum, DBFieldFloat, DBFieldInt, DBFieldSerialized, DBFieldSet, and DBFieldText.
Definition at line 96 of file dbfield.cls.php.
00096 { 00097 $ret = new Status(); 00098 if ($this->is_null($value) && !$this->has_default_value() && !$this->get_null_allowed()) { 00099 $field_tr = $this->get_field_name_translation(); 00100 $msg = tr('%field may not be empty', 'core', array('%field' => $field_tr)); 00101 $ret->append($msg); 00102 } 00103 return $ret; 00104 }
Member Data Documentation
DBField::$connection
[protected] |
Definition at line 41 of file dbfield.cls.php.
DBField::$default_value = null
[protected] |
Definition at line 29 of file dbfield.cls.php.
DBField::$name = ''
[protected] |
Definition at line 23 of file dbfield.cls.php.
DBField::$policy [protected] |
Definition at line 35 of file dbfield.cls.php.
DBField::$table [protected] |
Definition at line 47 of file dbfield.cls.php.
const DBField::INTERNAL = 8 |
Indicates this field is internal.
This flag can be checked when building UI or on updates
Definition at line 16 of file dbfield.cls.php.
const DBField::NOT_NULL = 1 |
Indicates this field may not be NULL.
Definition at line 12 of file dbfield.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbfield.cls.php