RouterBase Class Reference
[Controller]
The Router. More...
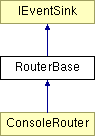
Public Member Functions |
|
__construct ($class_instantiater) | |
Constructor. |
|
get_route_id () | |
Returns the action invoked. |
|
initialize ($page_data) | |
Initialize page data. |
|
on_event ($name, $params, &$result) | |
Invoke event on all controllers. |
|
postprocess ($page_data) | |
Do what's necessary after page was
processed, e.g. |
|
preprocess ($page_data) | |
Prepares all controllers. |
|
route () | |
Looks for a handler of given url and invokes
it. |
|
Protected Member Functions |
|
find_route ($path) | |
Try to find matching controller. |
|
get_path () | |
Returns the current path, preprocessed.
|
|
Protected Attributes |
|
$controllers = array() | |
Array of controllers. |
|
$current_route = null |
Detailed Description
The Router.
Definition at line 10 of file routerbase.cls.php.
Constructor & Destructor Documentation
RouterBase::__construct | ( | $ | class_instantiater | ) |
Constructor.
Loads all controllers
Definition at line 28 of file routerbase.cls.php.
00028 { 00029 $potential_controllers = $class_instantiater->get_all(); 00030 foreach ($potential_controllers as $controller) { 00031 if ($controller instanceof IController) { 00032 $this->controllers[] = $controller; 00033 } 00034 } 00035 EventSource::Instance()->register($this); 00036 }
Member Function Documentation
RouterBase::find_route | ( | $ | path | ) | [protected] |
Try to find matching controller.
Definition at line 112 of file routerbase.cls.php.
00112 { 00113 if ($path === '') { 00114 return null; 00115 } 00116 $best_matching_route = null; 00117 $best_weight = IRoute::WEIGHT_NO_MATCH; 00118 00119 foreach ($this->controllers as $controller) { 00120 $routes = $controller->get_routes(); 00121 foreach ($routes as $route) { 00122 $weight = $route->weight_against_path($path); 00123 //print $route->identify() . ': ' . $weight . '..' . $best_weight . '<br />'; 00124 if ($weight < $best_weight) { 00125 $best_matching_route = $route; 00126 $best_weight = $weight; 00127 } 00128 } 00129 } 00130 00131 return $best_matching_route; 00132 }
RouterBase::get_path | ( | ) | [protected] |
Returns the current path, preprocessed.
If index page is invoked, '.' is returned
- Returns:
- string The current path, e.g. path/to/page
Reimplemented in ConsoleRouter.
Definition at line 141 of file routerbase.cls.php.
00141 { 00142 // Check if index.php was invoked directly 00143 //if (RequestInfo::current() 00144 // ->url_invoked(RequestInfo::RELATIVE) == Config::get_url(Config::URL_BASEDIR) . 'index.php' 00145 //) { 00146 // return ''; 00147 //} 00148 00149 //$path = Arr::get_item($_GET, Config::get_value(Config::QUERY_PARAM_PATH_INVOKED), '.'); 00150 00151 // Switching to Url::current solves both the problem of detecting "index.php" from above 00152 // and some strange behaviour on (F)CGI machines, where 00153 // http://www.example.com/blah%3Fa%3Db gets passed as http://www.example.com/blah?a=b 00154 // whereas at the same time, the SERVER array contains the (correct!) original %3Fa%3Db, 00155 // which leads to confusion and may cause duplicate content URLs. 00156 $path = Url::current()->get_path(); 00157 if ($path === '') { 00158 $path = '.'; 00159 } 00160 return $path; 00161 }
RouterBase::get_route_id | ( | ) |
Returns the action invoked.
Definition at line 81 of file routerbase.cls.php.
RouterBase::initialize | ( | $ | page_data | ) |
Initialize page data.
Definition at line 41 of file routerbase.cls.php.
00041 { 00042 $path = $this->get_path(); 00043 //if (String::ends_with($path, '/')) { 00044 // $path = trim($path, '/'); 00045 //} 00046 $this->path_invoked = $path; 00047 $page_data->set_path($this->path_invoked); 00048 $page_data->router = $this; 00049 }
RouterBase::on_event | ( | $ | name, | |
$ | params, | |||
&$ | result | |||
) |
Invoke event on all controllers.
Implements IEventSink.
Definition at line 166 of file routerbase.cls.php.
00166 { 00167 $ret = new Status(); 00168 foreach ($this->controllers as $controller) { 00169 $ret->merge($controller->on_event($name, $params, $result)); 00170 } 00171 return $ret; 00172 }
RouterBase::postprocess | ( | $ | page_data | ) |
Do what's necessary after page was processed, e.g.
create sidebar items...
Definition at line 103 of file routerbase.cls.php.
RouterBase::preprocess | ( | $ | page_data | ) |
Prepares all controllers.
- Parameters:
-
string Data of current page
Definition at line 94 of file routerbase.cls.php.
RouterBase::route | ( | ) |
Looks for a handler of given url and invokes it.
- Returns:
- IRoute
Definition at line 56 of file routerbase.cls.php.
00056 { 00057 $token = $this->find_route($this->path_invoked); 00058 if (empty($token)) { 00059 $token = new NotFoundRoute($this->path_invoked); 00060 } 00061 else { 00062 // SImualte Apache behaviout that a becomes a/ if a/ is defiend, but a not 00063 $path_current = Url::current()->get_path(); 00064 $current_is_dir = (substr($path_current, -1) === '/'); 00065 $route_is_dir = $token->is_directory(); 00066 00067 if ($route_is_dir && !$current_is_dir) { 00068 Url::current()->set_path($path_current . '/')->redirect(Url::PERMANENT); 00069 } 00070 else if (!$route_is_dir && $current_is_dir) { 00071 Url::current()->set_path(rtrim($path_current, '/'))->redirect(Url::PERMANENT); 00072 } 00073 } 00074 $this->current_route = $token; 00075 return $token; 00076 }
Member Data Documentation
RouterBase::$controllers = array()
[protected] |
Array of controllers.
Definition at line 16 of file routerbase.cls.php.
RouterBase::$current_route = null
[protected] |
Definition at line 23 of file routerbase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/controller/base/routerbase.cls.php