PageData Class Reference
[Controller]
Collects data used to render a page and to be exchange between different parts of the application. More...
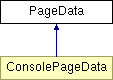
Public Member Functions |
|
__clone () | |
__construct ($cache_manager, $get, $post) | |
Constructor. |
|
add_block ($block, $position=false, $weight=false) | |
Add a block. |
|
add_render_decorator_class ($dec) | |
Add a render decorator to overload default
render behaviour. |
|
error ($msg) | |
Set error. |
|
get_blocks ($position=false) | |
Get blocks for given position. |
|
get_cache_manager () | |
Returns cache manager. |
|
get_get () | |
Return GET data. |
|
get_pathstack () | |
Returns path stack. |
|
get_post () | |
Return POST data. |
|
get_render_decorators ($route) | |
Returns array of render decorators to
overload default render behaviour. |
|
has_post_data () | |
Returns true if data has been posted.
|
|
message ($msg) | |
Set (success) message. |
|
raw_request_body () | |
Returns the raw data send along with a
request (for POST, PUT, DELETE). |
|
set_cache_manager ($cache_manager) | |
Set cache manager. |
|
set_path ($path) | |
Set current url path. |
|
sort_blocks () | |
Sort blocks. |
|
successful () | |
Returns true, if page call was successfull,
false otherwise (CONTROLLER_NOTFOUND et al). |
|
Public Attributes |
|
$blocks = array() | |
$breadcrumb | |
$content = '' | |
$head | |
$in_history = true | |
$page_template | |
Page template. |
|
$pathstack = null | |
$router = null | |
$status | |
$status_code = CONTROLLER_OK | |
Protected Attributes |
|
$cache_manager = null | |
$render_decorator_classes = array() |
Detailed Description
Collects data used to render a page and to be exchange between different parts of the application.
One instance of PageData is passed around in all steps of the rendering process.
Definition at line 12 of file pagedata.cls.php.
Constructor & Destructor Documentation
PageData::__construct | ( | $ | cache_manager, | |
$ | get, | |||
$ | post | |||
) |
Constructor.
- Parameters:
-
ICacheManager $cache_manager array $get Usually $_GET array $post Usually $_POST
Reimplemented in ConsolePageData.
Definition at line 103 of file pagedata.cls.php.
00103 { 00104 $this->page_template = Config::get_value(Config::PAGE_TEMPLATE); 00105 $this->status = Status::restore(); 00106 $this->head = new HeadData(); 00107 //if (empty($this->status)) { 00108 // $this->status = new Status(); 00109 //} 00110 if (empty($cache_manager)) { $cache_manager = new NoCacheCacheManager(); } 00111 $this->set_cache_manager($cache_manager); 00112 $this->pathstack = new PathStack(); 00113 00114 // GET array 00115 $tmp = Arr::force($get); 00116 array_walk_recursive($tmp, array($this, 'trim_array_content')); 00117 // No path param, since this defines url 00118 unset($tmp[Config::get_value(Config::QUERY_PARAM_PATH_INVOKED)]); 00119 $this->get = new TracedArray($tmp); 00120 00121 // POST array 00122 $tmp = Arr::force($post); 00123 array_walk_recursive($tmp, array($this, 'trim_array_content')); 00124 $this->post = new TracedArray($this->preprocess_post($tmp)); 00125 }
Member Function Documentation
PageData::__clone | ( | ) |
Definition at line 127 of file pagedata.cls.php.
00127 { 00128 // Force a copy of this->object, otherwise 00129 // it will point to same object. 00130 $this->cache_manager = clone($this->cache_manager); 00131 $this->get = clone($this->get); 00132 $this->post = clone($this->post); 00133 $this->head = clone($this->head); 00134 $this->pathstack = clone($this->pathstack); 00135 if ($this->status) { 00136 $this->status = clone($this->status); 00137 } 00138 }
PageData::add_render_decorator_class | ( | $ | dec | ) |
Add a render decorator to overload default render behaviour.
- Parameters:
-
string $dec Class name of render decorator
Definition at line 282 of file pagedata.cls.php.
PageData::error | ( | $ | msg | ) |
Set error.
- Parameters:
-
Status|string $msg Error or error message
Definition at line 365 of file pagedata.cls.php.
PageData::get_blocks | ( | $ | position = false |
) |
Get blocks for given position.
- Parameters:
-
string $position Pass FALSE to retrieve all blocks
Definition at line 349 of file pagedata.cls.php.
PageData::get_cache_manager | ( | ) |
PageData::get_get | ( | ) |
PageData::get_pathstack | ( | ) |
PageData::get_post | ( | ) |
PageData::get_render_decorators | ( | $ | route | ) |
Returns array of render decorators to overload default render behaviour.
- Parameters:
-
IDispatcher Dispatcher
- Returns:
- array Array of IRenderDecorators
Definition at line 292 of file pagedata.cls.php.
PageData::has_post_data | ( | ) |
Returns true if data has been posted.
- Returns:
- bool
Definition at line 241 of file pagedata.cls.php.
PageData::message | ( | $ | msg | ) |
Set (success) message.
- Parameters:
-
Message|string $msg Message instance or message
Definition at line 382 of file pagedata.cls.php.
00382 { 00383 if ($msg instanceof Message) { 00384 $this->status = $msg; 00385 } 00386 else { 00387 $this->status = new Message(strval($msg)); 00388 } 00389 }
PageData::raw_request_body | ( | ) |
Returns the raw data send along with a request (for POST, PUT, DELETE).
- Returns:
- string
Definition at line 250 of file pagedata.cls.php.
PageData::set_cache_manager | ( | $ | cache_manager | ) |
Set cache manager.
- Parameters:
-
ICacheManager $cache_manager
Definition at line 270 of file pagedata.cls.php.
00270 { 00271 if (!empty($cache_manager)) { 00272 $this->cache_manager = $cache_manager; 00273 $this->cache_manager->initialize($this); 00274 } 00275 }
PageData::set_path | ( | $ | path | ) |
PageData::sort_blocks | ( | ) |
Sort blocks.
Definition at line 338 of file pagedata.cls.php.
PageData::successful | ( | ) |
Returns true, if page call was successfull, false otherwise (CONTROLLER_NOTFOUND et al).
- Returns:
- bool
Definition at line 396 of file pagedata.cls.php.
00396 { 00397 return empty($this->status_code) || $this->status_code == CONTROLLER_OK; 00398 }
Member Data Documentation
PageData::$blocks = array() |
Definition at line 54 of file pagedata.cls.php.
PageData::$breadcrumb |
Definition at line 30 of file pagedata.cls.php.
PageData::$cache_manager = null
[protected] |
Definition at line 84 of file pagedata.cls.php.
PageData::$content = '' |
Definition at line 18 of file pagedata.cls.php.
PageData::$head |
Definition at line 24 of file pagedata.cls.php.
PageData::$in_history = true |
Definition at line 60 of file pagedata.cls.php.
PageData::$page_template |
Page template.
Definition at line 94 of file pagedata.cls.php.
PageData::$pathstack = null |
Definition at line 48 of file pagedata.cls.php.
PageData::$render_decorator_classes = array()
[protected] |
Definition at line 90 of file pagedata.cls.php.
PageData::$router = null |
Definition at line 66 of file pagedata.cls.php.
PageData::$status |
Definition at line 36 of file pagedata.cls.php.
PageData::$status_code = CONTROLLER_OK |
Definition at line 42 of file pagedata.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/controller/base/pagedata.cls.php