DBTable Class Reference
[Model]
Represents a DB table. More...
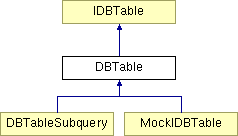
Public Member Functions |
|
__construct ($name, $fields=null, $keys=null, $relations=null, $constraints=null, $driver=null) | |
add_constraint (IDBConstraint $constraint) | |
Adds a constraint to the collection of
constraints. |
|
add_field (IDBField $field) | |
Adds a field to the collection of fields.
|
|
add_relation (IDBRelation $relation) | |
Adds a relation to the collection of
relations. |
|
get_matching_relations (IDBTable $other) | |
Returns relations between two tables.
|
|
get_table_alias () | |
Returns alias of table, if any. |
|
get_table_alias_escaped () | |
Returns alias of table, if any - but
escaped. |
|
get_table_constraints () | |
Returns array of constraints. |
|
get_table_driver () | |
Returns DB driver fro this
table. |
|
get_table_field ($column) | |
Returns field fpr given column. |
|
get_table_fields () | |
Returns array of columns. |
|
get_table_keys () | |
Returns array of keys. |
|
get_table_name () | |
Returns name of table. |
|
get_table_name_escaped () | |
Returns name of table, but escaped. |
|
get_table_relations () | |
Returns array of relations. |
|
set_as_key ($column) | |
Define column $column as key. |
|
Protected Member Functions |
|
find_relations (IDBTable $source, IDBTable $target) | |
Find relations between source and target.
|
|
relation_is_in_array (IDBRelation $relation, $arr_relations) | |
Returns true, if there is a counterpart for
given relation in array of others. |
|
remove_duplicated_relations ($arr1, $arr2) | |
Returns relations from arr1 that are not
contained in arr2. |
|
Protected Attributes |
|
$alias = '' | |
$constraints = array() | |
$driver | |
$fields = array() | |
$keys = array() | |
$name = '' | |
$relations = array() |
Detailed Description
Represents a DB table.
Definition at line 8 of file dbtable.cls.php.
Constructor & Destructor Documentation
DBTable::__construct | ( | $ | name, | |
$ | fields = null , |
|||
$ | keys = null , |
|||
$ | relations = null , |
|||
$ | constraints =
null , |
|||
$ | driver = null |
|||
) |
Definition at line 52 of file dbtable.cls.php.
00052 { 00053 $this->driver = empty($driver) ? DB::get_connection(DB::DEFAULT_CONNECTION) : DB::get_connection($driver); 00054 $this->name = $name; 00055 $this->alias = $name; 00056 if (is_array($fields)) { 00057 foreach($fields as $field) { 00058 $this->add_field($field); 00059 } 00060 } 00061 if (!empty($keys)) { 00062 foreach(Arr::force($keys) as $key) { 00063 $this->set_as_key($key); 00064 } 00065 } 00066 if (!empty($relations)) { 00067 foreach(Arr::force($relations) as $relation) { 00068 $this->add_relation($relation); 00069 } 00070 } 00071 if (!empty($constraints)) { 00072 foreach(Arr::force($constraints) as $constraint) { 00073 $this->add_constraint($constraint); 00074 } 00075 } 00076 }
Member Function Documentation
DBTable::add_constraint | ( | IDBConstraint $ | constraint | ) |
Adds a constraint to the collection of constraints.
- Parameters:
-
IDBConstraint $constraint
Definition at line 290 of file dbtable.cls.php.
DBTable::add_field | ( | IDBField $ | field | ) |
Adds a field to the collection of fields.
- Parameters:
-
IDBField $field
Definition at line 270 of file dbtable.cls.php.
DBTable::add_relation | ( | IDBRelation $ | relation | ) |
Adds a relation to the collection of relations.
- Parameters:
-
IDBRelation $relation
Definition at line 281 of file dbtable.cls.php.
Find relations between source and target.
- Returns:
- array Array of IDBRelations
Definition at line 189 of file dbtable.cls.php.
DBTable::get_matching_relations | ( | IDBTable $ | other | ) |
Returns relations between two tables.
- Parameters:
-
IDBTable $other
- Returns:
- array Array of IDBRelations
Implements IDBTable.
Definition at line 169 of file dbtable.cls.php.
00169 { 00170 $relations_this2other = $this->find_relations($this, $other); 00171 $relations_other2this = $this->find_relations($other, $this); 00172 00173 $relations_other2this = $this->remove_duplicated_relations($relations_other2this, $relations_this2other); 00174 00175 foreach($relations_other2this as $relation) { 00176 $relations_this2other[] = new DBRelation($other->get_table_name(), $relation->get_reversed_fields(), $relation->get_policy(), $relation->get_type()); 00177 } 00178 00179 return $relations_this2other; 00180 }
DBTable::get_table_alias | ( | ) |
Returns alias of table, if any.
- Returns:
- string
Implements IDBTable.
Definition at line 94 of file dbtable.cls.php.
DBTable::get_table_alias_escaped | ( | ) |
Returns alias of table, if any - but escaped.
- Returns:
- string
Implements IDBTable.
Definition at line 112 of file dbtable.cls.php.
00112 { 00113 return $this->driver->escape_database_entity($this->alias, IDBDriver::ALIAS); 00114 }
DBTable::get_table_constraints | ( | ) |
Returns array of constraints.
- Returns:
- array Array with IDBConstraint instance as value
Implements IDBTable.
Definition at line 257 of file dbtable.cls.php.
DBTable::get_table_driver | ( | ) |
Returns DB driver fro this table.
- Returns:
- IDBDriver
Implements IDBTable.
Definition at line 150 of file dbtable.cls.php.
DBTable::get_table_field | ( | $ | column | ) |
Returns field fpr given column.
- Parameters:
-
string $column Column name
- Returns:
- IDBField Either field or false if no such field exists
Implements IDBTable.
Definition at line 131 of file dbtable.cls.php.
00131 { 00132 $ret = Arr::get_item($this->fields, $column, false); 00133 return $ret; 00134 }
DBTable::get_table_fields | ( | ) |
Returns array of columns.
- Returns:
- array Associative array with column name as key and IDBField instance as value
Implements IDBTable.
Definition at line 121 of file dbtable.cls.php.
DBTable::get_table_keys | ( | ) |
Returns array of keys.
- Returns:
- array Associative array with column name as key and IDField instance as value
Implements IDBTable.
Definition at line 141 of file dbtable.cls.php.
DBTable::get_table_name | ( | ) |
DBTable::get_table_name_escaped | ( | ) |
Returns name of table, but escaped.
- Returns:
- string
Implements IDBTable.
Reimplemented in DBTableSubquery.
Definition at line 103 of file dbtable.cls.php.
00103 { 00104 return $this->driver->escape_database_entity($this->name, IDBDriver::TABLE); 00105 }
DBTable::get_table_relations | ( | ) |
Returns array of relations.
- Returns:
- array Array with IDBRelation instance as value
Implements IDBTable.
Definition at line 159 of file dbtable.cls.php.
DBTable::relation_is_in_array | ( | IDBRelation $ | relation, | |
$ | arr_relations | |||
) | [protected] |
Returns true, if there is a counterpart for given relation in array of others.
- Parameters:
-
IDBRelation $relation array $arr_relations
- Returns:
- bool
Definition at line 224 of file dbtable.cls.php.
00224 { 00225 $duplicate = false; 00226 // These are the fields 00227 $fields_to_check = array(); 00228 foreach($relation->get_fields() as $fieldrelation) { 00229 $fields_to_check[$fieldrelation->get_source_field_name()] = $fieldrelation->get_target_field_name(); 00230 } 00231 $c_check = count($fields_to_check); 00232 foreach($arr_relations as $relation_to_test_against) { 00233 $fields = $relation_to_test_against->get_fields(); 00234 if (count($fields) != $c_check) { 00235 continue; 00236 } 00237 $duplicate = true; 00238 foreach($fields as $fieldrelation) { 00239 $fieldname_to_check = Arr::get_item($fields_to_check, $fieldrelation->get_target_field_name(), ''); 00240 if ($fieldname_to_check != $fieldrelation->get_source_field_name()) { 00241 $duplicate = false; 00242 break; 00243 } 00244 } 00245 if ($duplicate) { 00246 break; 00247 } 00248 } 00249 return $duplicate; 00250 }
DBTable::remove_duplicated_relations | ( | $ | arr1, | |
$ | arr2 | |||
) | [protected] |
Returns relations from arr1 that are not contained in arr2.
- Parameters:
-
array $arr1 Array of IDBRelations array $arr2 Array of IDBRelations
- Returns:
- array Array of IDBRelations
Definition at line 207 of file dbtable.cls.php.
00207 { 00208 $ret = array(); 00209 foreach($arr1 as $parent_relation) { 00210 if (!$this->relation_is_in_array($parent_relation, $arr2)) { 00211 $ret[] = $parent_relation; 00212 } 00213 } 00214 return $ret; 00215 }
DBTable::set_as_key | ( | $ | column | ) |
Define column $column as key.
- Parameters:
-
string $column
Definition at line 299 of file dbtable.cls.php.
00299 { 00300 $key_field = $this->get_table_field($column); 00301 if ($key_field) { 00302 $this->keys[$column] = $key_field; 00303 } 00304 else { 00305 throw new Exception(tr('Can not set key - no field %col', 'core', array('%col' => $column))); 00306 } 00307 }
Member Data Documentation
DBTable::$alias = ''
[protected] |
Definition at line 20 of file dbtable.cls.php.
DBTable::$constraints = array()
[protected] |
Definition at line 44 of file dbtable.cls.php.
DBTable::$driver [protected] |
Definition at line 50 of file dbtable.cls.php.
DBTable::$fields = array()
[protected] |
Definition at line 26 of file dbtable.cls.php.
DBTable::$keys = array()
[protected] |
Definition at line 32 of file dbtable.cls.php.
DBTable::$name = ''
[protected] |
Definition at line 14 of file dbtable.cls.php.
DBTable::$relations = array()
[protected] |
Definition at line 38 of file dbtable.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbtable.cls.php