DBSortColumn Class Reference
[Model]
A sortable column. More...
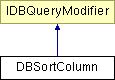
Public Member Functions |
|
__construct ($column, $title, $type, $direction=self::ORDER_FORWARD, $single_direction=false) | |
contructor |
|
apply ($query) | |
Do what should be done. |
|
get_column () | |
get_direction () | |
get_is_single_direction () | |
Returns true if this column should be sorted
in a single direction only. |
|
get_opposite_order ($direction) | |
Return reverse direction. |
|
get_order_title ($direction) | |
Return title for sort direction. |
|
get_sort_order ($direction) | |
Return sort direction key for sorting.
|
|
get_title () | |
set_direction ($direction) | |
Public Attributes |
|
const | ORDER_BACKWARD = 'backward' |
const | ORDER_FORWARD = 'forward' |
const | TYPE_CURRENCY = 'currency' |
const | TYPE_DATE = 'date' |
const | TYPE_MATCH = 'match' |
const | TYPE_NUMERIC = 'numeric' |
const | TYPE_TEXT = 'text' |
Protected Member Functions |
|
get_db_column () | |
Returns name of column in DB.
|
Detailed Description
A sortable column.
Definition at line 8 of file dbsortcolumn.cls.php.
Constructor & Destructor Documentation
DBSortColumn::__construct | ( | $ | column, | |
$ | title, | |||
$ | type, | |||
$ | direction =
self::ORDER_FORWARD , |
|||
$ | single_direction = false |
|||
) |
contructor
- Parameters:
-
string column string title enum type of column
Definition at line 48 of file dbsortcolumn.cls.php.
Member Function Documentation
DBSortColumn::apply | ( | $ | query | ) |
Do what should be done.
- Parameters:
-
DataObjectBase The current query to be modified
Implements IDBQueryModifier.
Definition at line 56 of file dbsortcolumn.cls.php.
00056 { 00057 $query->sort($this->get_db_column(), $this->get_sort_order($this->direction)); 00058 }
DBSortColumn::get_column | ( | ) |
Definition at line 69 of file dbsortcolumn.cls.php.
DBSortColumn::get_db_column | ( | ) | [protected] |
Returns name of column in DB.
- Returns:
- unknown
Definition at line 65 of file dbsortcolumn.cls.php.
00065 { 00066 return $this->get_column(); 00067 }
DBSortColumn::get_direction | ( | ) |
Definition at line 92 of file dbsortcolumn.cls.php.
DBSortColumn::get_is_single_direction | ( | ) |
Returns true if this column should be sorted in a single direction only.
- Returns:
- true
Definition at line 82 of file dbsortcolumn.cls.php.
DBSortColumn::get_opposite_order | ( | $ | direction | ) |
Return reverse direction.
Definition at line 162 of file dbsortcolumn.cls.php.
DBSortColumn::get_order_title | ( | $ | direction | ) |
Return title for sort direction.
Definition at line 99 of file dbsortcolumn.cls.php.
00099 { 00100 if ($direction == self::ORDER_FORWARD) { 00101 switch ($this->type) { 00102 case self::TYPE_CURRENCY: 00103 return tr('Ascending (cheaper first)', 'core'); 00104 break; 00105 case self::TYPE_DATE: 00106 return tr('Ascending (newer first)', 'core'); 00107 break; 00108 case self::TYPE_TEXT: 00109 return tr('Ascending (A-Z)', 'core'); 00110 break; 00111 case self::TYPE_MATCH: 00112 return tr('Ascending (Most important first)', 'core'); 00113 break; 00114 case self::TYPE_NUMERIC: 00115 default: 00116 return tr('Ascending (smaller first)', 'core'); 00117 break; 00118 } 00119 } 00120 else { 00121 switch ($this->type) { 00122 case self::TYPE_CURRENCY: 00123 return tr('Descending (expensive first)', 'core'); 00124 break; 00125 case self::TYPE_DATE: 00126 return tr('Descending (older first)', 'core'); 00127 break; 00128 case self::TYPE_TEXT: 00129 return tr('Descending (Z-A)', 'core'); 00130 break; 00131 case self::TYPE_MATCH: 00132 return tr('Descending (Less important first)', 'core'); 00133 break; 00134 case self::TYPE_NUMERIC: 00135 default: 00136 return tr('Descending (greater first)', 'core'); 00137 break; 00138 } 00139 } 00140 }
DBSortColumn::get_sort_order | ( | $ | direction | ) |
Return sort direction key for sorting.
This class supports forward and backward sorting. Translate this into ascending/descending
Definition at line 147 of file dbsortcolumn.cls.php.
00147 { 00148 switch ($this->type) { 00149 case self::TYPE_DATE: 00150 case self::TYPE_MATCH: 00151 return ($direction == self::ORDER_BACKWARD) ? ISearchAdapter::ASC : ISearchAdapter::DESC; 00152 break; 00153 default: 00154 return ($direction == self::ORDER_BACKWARD) ? ISearchAdapter::DESC : ISearchAdapter::ASC; 00155 break; 00156 } 00157 }
DBSortColumn::get_title | ( | ) |
Definition at line 73 of file dbsortcolumn.cls.php.
DBSortColumn::set_direction | ( | $ | direction | ) |
Definition at line 86 of file dbsortcolumn.cls.php.
00086 { 00087 if (!$this->get_is_single_direction()) { 00088 $this->direction = $direction; 00089 } 00090 }
Member Data Documentation
const DBSortColumn::ORDER_BACKWARD = 'backward' |
Definition at line 16 of file dbsortcolumn.cls.php.
const DBSortColumn::ORDER_FORWARD = 'forward' |
Definition at line 15 of file dbsortcolumn.cls.php.
const DBSortColumn::TYPE_CURRENCY = 'currency' |
Definition at line 10 of file dbsortcolumn.cls.php.
const DBSortColumn::TYPE_DATE = 'date' |
Definition at line 12 of file dbsortcolumn.cls.php.
const DBSortColumn::TYPE_MATCH = 'match' |
Definition at line 13 of file dbsortcolumn.cls.php.
const DBSortColumn::TYPE_NUMERIC = 'numeric' |
Definition at line 11 of file dbsortcolumn.cls.php.
const DBSortColumn::TYPE_TEXT = 'text' |
Definition at line 9 of file dbsortcolumn.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbsortcolumn.cls.php