DBQuerySelect Class Reference
[Model]
A select query. More...
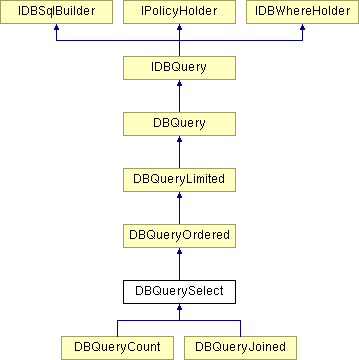
Public Member Functions |
|
__construct ($table, $policy=self::NORMAL) | |
add_group_by ($column) | |
Add group by, without any column the current
group by is cleared. |
|
add_having ($column, $operator=null, $value=null, $mode=IDBWhere::LOGIC_AND) | |
Adds having clause to this. |
|
add_having_object (IDBWhere $having) | |
Adds IDBWhere instance
to this. |
|
add_join (IDBTable $table, $policy=DBQueryJoined::AUTODETECT_CONDITIONS, $join_type=DBQueryJoined::INNER) | |
Add a table to join. |
|
add_subquery (DBQuerySelect $query) | |
Add a sub query (this is: a join). |
|
get_group_bys () | |
Returns group by. |
|
get_havings () | |
Returns root collection of having. |
|
get_sql () | |
Return SQL fragment. |
|
get_subqueries () | |
Return sub queries. |
|
get_wheres () | |
Returns collection of wheres. |
|
Public Attributes |
|
const | DISTINCT = 1 |
const | FOR_UPDATE = 2 |
Protected Member Functions |
|
create_sql_builder ($params) | |
Create SQL builder. |
|
prepare_sql_params () | |
Prepare params for SQL Builder. |
|
Protected Attributes |
|
$group_bys = array() | |
$havings | |
$subqueries = array() |
Detailed Description
A select query.
Definition at line 10 of file dbquery.select.cls.php.
Constructor & Destructor Documentation
DBQuerySelect::__construct | ( | $ | table, | |
$ | policy =
self::NORMAL |
|||
) |
Definition at line 33 of file dbquery.select.cls.php.
00033 { 00034 parent::__construct($table, $policy); 00035 $this->havings = new DBWhereGroup($table); 00036 }
Member Function Documentation
DBQuerySelect::add_group_by | ( | $ | column | ) |
Add group by, without any column the current group by is cleared.
- Parameters:
-
string $column
Definition at line 139 of file dbquery.select.cls.php.
DBQuerySelect::add_having | ( | $ | column, | |
$ | operator = null , |
|||
$ | value = null , |
|||
$ | mode = IDBWhere::LOGIC_AND |
|||
) |
Adds having clause to this.
- Parameters:
-
string $column Column to query upon, or a full sql where statement string $operator Operator to execute mixed $value Value(s) to use string $mode Either IDBWhere::LOGIC_AND or IDBWhere::LOGIC_OR
Definition at line 168 of file dbquery.select.cls.php.
DBQuerySelect::add_having_object | ( | IDBWhere $ | having | ) |
Adds IDBWhere instance to this.
Definition at line 175 of file dbquery.select.cls.php.
DBQuerySelect::add_join | ( | IDBTable $ | table, | |
$ | policy = DBQueryJoined::AUTODETECT_CONDITIONS , |
|||
$ | join_type = DBQueryJoined::INNER |
|||
) |
Add a table to join.
- Parameters:
-
IDBTable $table int $join_type One of the constants defined in DBQueryJoined
- Returns:
- DBQueryJoined
Definition at line 88 of file dbquery.select.cls.php.
00088 { 00089 $join = new DBQueryJoined($table, $this, $join_type, $policy); 00090 $this->add_subquery($join); 00091 return $join; 00092 }
DBQuerySelect::add_subquery | ( | DBQuerySelect $ | query | ) |
Add a sub query (this is: a join).
- Parameters:
-
DBQuerySelect $query
Definition at line 108 of file dbquery.select.cls.php.
DBQuerySelect::create_sql_builder | ( | $ | params | ) | [protected] |
Create SQL builder.
- Parameters:
-
array $params
- Returns:
- IDBSqlBuilder
Reimplemented in DBQueryCount.
Definition at line 55 of file dbquery.select.cls.php.
00055 { 00056 $builder = DBSqlBuilderFactory::create_builder(DBSqlBuilderFactory::SELECT, $this, $params); 00057 return $builder; 00058 }
DBQuerySelect::get_group_bys | ( | ) |
Returns group by.
- Returns:
- array Array of associative arrays of form "field => ..., table => ..."
Definition at line 156 of file dbquery.select.cls.php.
DBQuerySelect::get_havings | ( | ) |
Returns root collection of having.
- Returns:
- DBWhereGroup
Definition at line 131 of file dbquery.select.cls.php.
DBQuerySelect::get_sql | ( | ) |
Return SQL fragment.
- Returns:
- string
Implements IDBSqlBuilder.
Definition at line 43 of file dbquery.select.cls.php.
00043 { 00044 $params = $this->prepare_sql_params(); 00045 $builder = $this->create_sql_builder($params); 00046 return $builder->get_sql(); 00047 }
DBQuerySelect::get_subqueries | ( | ) |
DBQuerySelect::get_wheres | ( | ) |
Returns collection of wheres.
- Returns:
- DBWhereGroup
Reimplemented from DBQuery.
Definition at line 117 of file dbquery.select.cls.php.
00117 { 00118 $ret = new DBWhereGroup($this->get_table()); 00119 $ret->add_where_object(parent::get_wheres()); 00120 foreach($this->subqueries as $subquery) { 00121 $ret->add_where_object($subquery->get_wheres()); 00122 } 00123 return $ret; 00124 }
DBQuerySelect::prepare_sql_params | ( | ) | [protected] |
Prepare params for SQL Builder.
- Returns:
- array
Definition at line 65 of file dbquery.select.cls.php.
00065 { 00066 $params = array(); 00067 if ($this->policy & self::DISTINCT) { 00068 $params['distinct'] = true; 00069 } 00070 if ($this->policy & self::FOR_UPDATE) { 00071 $params['for_update'] = true; 00072 } 00073 $params['fields'] = $this->fields; 00074 $params['group_by'] = $this->get_group_bys(); 00075 $params['having'] = $this->get_havings(); 00076 $params['limit'] = $this->get_limit(); 00077 $params['order_by'] = $this->get_orders(); 00078 return $params; 00079 }
Member Data Documentation
DBQuerySelect::$group_bys = array()
[protected] |
Definition at line 25 of file dbquery.select.cls.php.
DBQuerySelect::$havings
[protected] |
Definition at line 31 of file dbquery.select.cls.php.
DBQuerySelect::$subqueries = array()
[protected] |
Definition at line 19 of file dbquery.select.cls.php.
const DBQuerySelect::DISTINCT = 1 |
Definition at line 11 of file dbquery.select.cls.php.
const DBQuerySelect::FOR_UPDATE = 2 |
Definition at line 12 of file dbquery.select.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/queries/dbquery.select.cls.php