CommandBase Class Reference
[Behaviour]
Base implementation for commands. More...
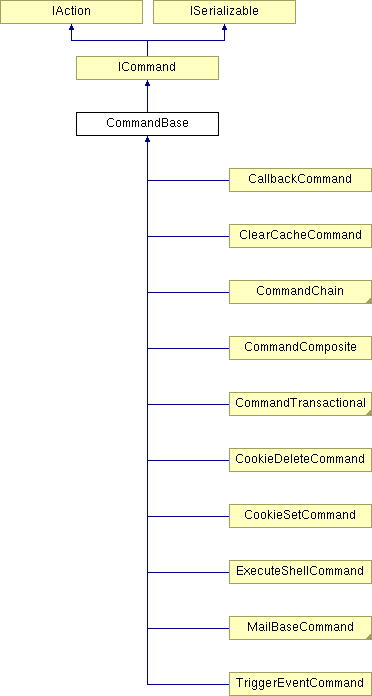
Public Member Functions |
|
__construct ($obj=null, $params=false) | |
can_execute ($user) | |
Returns TRUE, if the command can be executed
by given user. |
|
execute () | |
Executes commands. |
|
get_description () | |
Returns a description of this command.
|
|
get_instance () | |
Returns the object this actionworks upon.
|
|
get_name () | |
Returns title of command. |
|
get_name_serialized () | |
Returns a name that has parameters build in.
|
|
get_params () | |
Returns params. |
|
get_result () | |
Return result of command. |
|
get_success_message () | |
Returns success message for this command.
|
|
serialize () | |
Make this command available for text
processing systems (that is: the HTML code). |
|
undo () | |
Revert execution. |
|
Protected Member Functions |
|
illegal_data_error () | |
Create an illegal data Status class. |
|
serialize_instance_name ($inst) | |
serialize_params ($params) | |
set_result ($result) | |
Set result. |
|
Protected Attributes |
|
$obj | |
$params | |
Parameters for command. |
|
$result = false |
Detailed Description
Base implementation for commands.
Definition at line 11 of file commandbase.cls.php.
Constructor & Destructor Documentation
CommandBase::__construct | ( | $ | obj = null , |
|
$ | params = false |
|||
) |
Reimplemented in CallbackCommand, ExecuteSqlCommand, and ExecuteSqlScriptCommand.
Definition at line 31 of file commandbase.cls.php.
Member Function Documentation
CommandBase::can_execute | ( | $ | user | ) |
Returns TRUE, if the command can be executed by given user.
Implements ICommand.
Reimplemented in CommandChain, CommandComposite, MailBaseCommand, and TriggerEventCommand.
Definition at line 43 of file commandbase.cls.php.
00043 { 00044 $name = $this->get_name(); 00045 $ret = true; 00046 if (!empty($name)) { 00047 $ret = AccessControl::is_allowed($name, $this->get_instance(), $this->get_params(), $user); 00048 } 00049 return $ret; 00050 }
CommandBase::execute | ( | ) |
Executes commands.
- Returns:
- Status
Implements ICommand.
Reimplemented in CommandChain, CommandComposite, CommandTransactional, MailBaseCommand, CallbackCommand, ClearCacheCommand, CookieDeleteCommand, CookieSetCommand, ExecuteShellCommand, and TriggerEventCommand.
Definition at line 57 of file commandbase.cls.php.
00057 { 00058 $ret = new Status(); 00059 EventSource::Instance()->invoke_event('command_executed', $this, $ret); 00060 return $ret; 00061 }
CommandBase::get_description | ( | ) |
Returns a description of this command.
Implements IAction.
Reimplemented in CreateCommand, DeleteCommand, UpdateCommand, and StatusAnyCommand.
Definition at line 80 of file commandbase.cls.php.
00080 { 00081 return $this->get_name(); 00082 }
CommandBase::get_instance | ( | ) |
Returns the object this actionworks upon.
- Returns:
- mixed
Implements IAction.
Definition at line 89 of file commandbase.cls.php.
CommandBase::get_name | ( | ) |
Returns title of command.
Implements IAction.
Reimplemented in CallbackCommand, CookieDeleteCommand, CookieSetCommand, CreateCommand, DeleteCommand, UpdateCommand, and StatusAnyCommand.
Definition at line 73 of file commandbase.cls.php.
CommandBase::get_name_serialized | ( | ) |
Returns a name that has parameters build in.
- Returns:
- string
Implements ICommand.
Definition at line 141 of file commandbase.cls.php.
00141 { 00142 $arr = array( 00143 $this->get_name() 00144 ); 00145 $params = $this->serialize_params($this->get_params()); 00146 if ($params) { 00147 $arr[] = $params; 00148 } 00149 return implode(GYRO_COMMAND_SEP, $arr); 00150 }
CommandBase::get_params | ( | ) |
Returns params.
- Returns:
- mixed
Implements ICommand.
Reimplemented in StatusAnyCommand.
Definition at line 98 of file commandbase.cls.php.
CommandBase::get_result | ( | ) |
Return result of command.
- Returns:
- mixed
Implements ICommand.
Definition at line 114 of file commandbase.cls.php.
CommandBase::get_success_message | ( | ) |
Returns success message for this command.
Implements ICommand.
Definition at line 105 of file commandbase.cls.php.
CommandBase::illegal_data_error | ( | ) | [protected] |
CommandBase::serialize | ( | ) |
Make this command available for text processing systems (that is: the HTML code).
The code returned looks like this: cmd_{instance type}_{instance id,[instance 2nd id, ..]}_{command name}_{command param}[_{command param}...]
Implements ISerializable.
Definition at line 127 of file commandbase.cls.php.
00127 { 00128 $arr = array( 00129 'cmd', 00130 $this->serialize_instance_name($this->get_instance()), 00131 $this->get_name_serialized() 00132 ); 00133 return implode(GYRO_COMMAND_SEP, $arr); 00134 }
CommandBase::serialize_instance_name | ( | $ | inst | ) | [protected] |
Definition at line 153 of file commandbase.cls.php.
00153 { 00154 $ret = ''; 00155 if ($inst instanceof IDataObject) { 00156 $arr = array( 00157 $inst->get_table_name() 00158 ); 00159 $arr_id = array(); 00160 foreach ($inst->get_table_keys() as $key => $field) { 00161 $arr_id[] = $inst->$key; 00162 } 00163 $arr[] = implode(GYRO_COMMAND_ID_SEP, $arr_id); 00164 $ret = implode(GYRO_COMMAND_SEP, $arr); 00165 } 00166 else { 00167 $ret = Cast::string($inst); 00168 } 00169 return $ret; 00170 }
CommandBase::serialize_params | ( | $ | params | ) | [protected] |
Definition at line 172 of file commandbase.cls.php.
00172 { 00173 $ret = ''; 00174 if (is_array($params)) { 00175 $arr = array(); 00176 foreach($params as $key => $value) { 00177 $arr[] = $key; 00178 $arr[] = $value; 00179 } 00180 $ret = implode(GYRO_COMMAND_SEP, $arr); 00181 } 00182 else { 00183 $ret = Cast::string($params); 00184 } 00185 return $ret; 00186 }
CommandBase::set_result | ( | $ | result | ) | [protected] |
Set result.
- Parameters:
-
mixed $result
Definition at line 197 of file commandbase.cls.php.
00197 { 00198 $this->result = $result; 00199 }
CommandBase::undo | ( | ) |
Revert execution.
Implements ICommand.
Reimplemented in CommandChain, and CommandComposite.
Definition at line 66 of file commandbase.cls.php.
Member Data Documentation
CommandBase::$obj [protected] |
Definition at line 17 of file commandbase.cls.php.
CommandBase::$params
[protected] |
CommandBase::$result = false
[protected] |
Definition at line 29 of file commandbase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/behaviour/base/commandbase.cls.php